What is CSS?
Cascading Style Sheets, often abbreviated as CSS, is a stylesheet language used to describe the look and formatting of a document written in HTML. While HTML structures the content and layout of a webpage, CSS is used to style this content - changing colors, fonts, spacing, and positioning.
Consider a house: HTML is the construction materials and the blueprint, while CSS is the paint, curtains, and decorative elements that make it look appealing.
Here's a basic example of how CSS is used:
<!DOCTYPE html> <html> <head> <style> body { background-color: powderblue; } h1 { color: blue; } p { color: red; } </style> </head> <body> <h1>This is a heading</h1> <p>This is a paragraph.</p> </body> </html>
This code will render the following way when you view it in a browser:
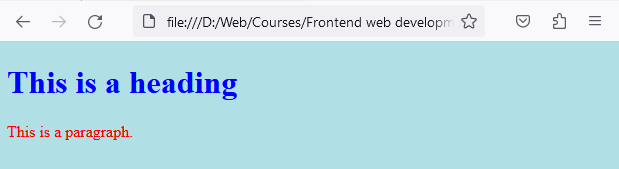
Why Use CSS?
CSS allows you to apply different styles to different elements in your HTML document, enhancing the overall look and feel. It gives you control over the visual presentation of your site and helps to separate the design from the content. This separation is beneficial as it improves site maintenance, ensures consistency, and allows for site-wide changes with minimal effort.
Basic CSS Syntax
A CSS rule-set consists of a selector and a declaration block. The selector points to the HTML element you want to style, and the declaration block contains one or more declarations separated by semicolons. Each declaration includes a CSS property name and a value, separated by a colon:
selector { property: value; }
Let's see examples of inline, internal, and external CSS.
Applying CSS to HTML
There are three ways you can apply CSS to an HTML document:
- Inline CSS: With this method, you use the style attribute directly within an HTML tag to apply CSS. However, inline styles have a high specificity and can override other CSS declarations in your stylesheets. It's generally best to use inline styles sparingly and for unique, one-off changes.
- Internal CSS: This involves placing CSS rules within <style> tags in the HTML document's <head> section. While this is a step up from inline styles, it can still lead to maintenance issues if your stylesheets become large and complex.
- External CSS: This is the most common way to apply CSS. Styles are placed in a separate .css file which is then linked to the HTML document using the <link> element. This method allows for clean, easy-to-manage code and is excellent for applying styles across multiple pages.
Inline CSS
Inline CSS is used to apply a unique style to a single HTML element. It uses the style attribute of an HTML element. This method of insertion applies the CSS rules directly into the HTML file.
An example of inline CSS is as follows:
<p style="color:blue;">This is a paragraph with inline CSS.</p>

However, inline CSS is rarely used because it mixes HTML with CSS making the document harder to read and maintain. It also doesn't leverage the advantage of CSS being able to control multiple elements at once.
Internal CSS
Internal CSS, also known as embedded CSS, is used to apply CSS to a whole HTML page. The CSS rules are put in a <style> block in the <head> section of the HTML file.
An example of internal CSS is as follows:
<!DOCTYPE html> <html> <head> <style> p { color: red; } </style> </head> <body> <p>This is a paragraph with internal CSS.</p> </body> </html>
- following will be rendered in the browser:

In this case, all the <p> tags on the page would be styled according to the CSS rules inside the <style> tags. Internal CSS is more manageable than inline CSS but still doesn't provide the reusability of external CSS.
External CSS
External CSS involves creating a separate .css file and linking it to your HTML document. This method of insertion aids in minimizing code redundancy and promotes reusability. A single external stylesheet can control the appearance of multiple HTML pages, making it easier to maintain and modify the styling of numerous pages at once.
Here's an example of how to link to an external CSS file:
1. First create an external CSS file inside your project folder (style.css)
p { color: green; }
2. Then, link this CSS file to your HTML document.
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="style.css"> </head> <body> <p>This is a paragraph with external CSS.</p> </body> </html>

CSS Comments
Comments in CSS are used to explain your code and can help you and others understand the purpose of the code. All CSS comments start with /* and end with */, and anything between these will be ignored by the browser.
<style> /* This is a single-line comment */ /* This is a multi-line comment */ </style>
Resetting Default CSS
Web browsers have their own default CSS styles for HTML elements, leading to inconsistencies across different browsers. These default styles are automatically applied in the absence of developer-defined styles and can affect the layout and design of your webpages unexpectedly.
Default browser styles can cause elements like headings, paragraphs, and lists to appear differently than intended, disrupting design uniformity across various browsers. To ensure consistency and start with a clean slate, developers use a CSS Reset.
A CSS Reset neutralizes all the default styles, providing a uniform, bare-bones styling baseline across all browsers.
/* Basic CSS Reset */ html, body, h1, h2, h3, h4, h5, h6, p, ol, ul, li, img, table, tr, td, th, input, textarea, button { margin: 0; padding: 0; border: 0; font-size: 100%; vertical-align: baseline; }
This example is a simple reset and is by no means exhaustive. Some developers prefer more comprehensive reset scripts, like Eric Meyer’s “Reset CSS” or “Normalize.css,” which preserves useful defaults rather than "unstyling" everything.
The mentioned CSS resets can be found on the following web pages:
Reset CSS: https://meyerweb.com/eric/tools/css/reset/
Normalize CSS: https://necolas.github.io/normalize.css/